Automated regression testing is a cornerstone for maintaining a high standard of quality in software development. Learn how to implement this crucial practice in this article, from defining objectives selecting the right tools to interpret results, and fine-tuning your strategy.
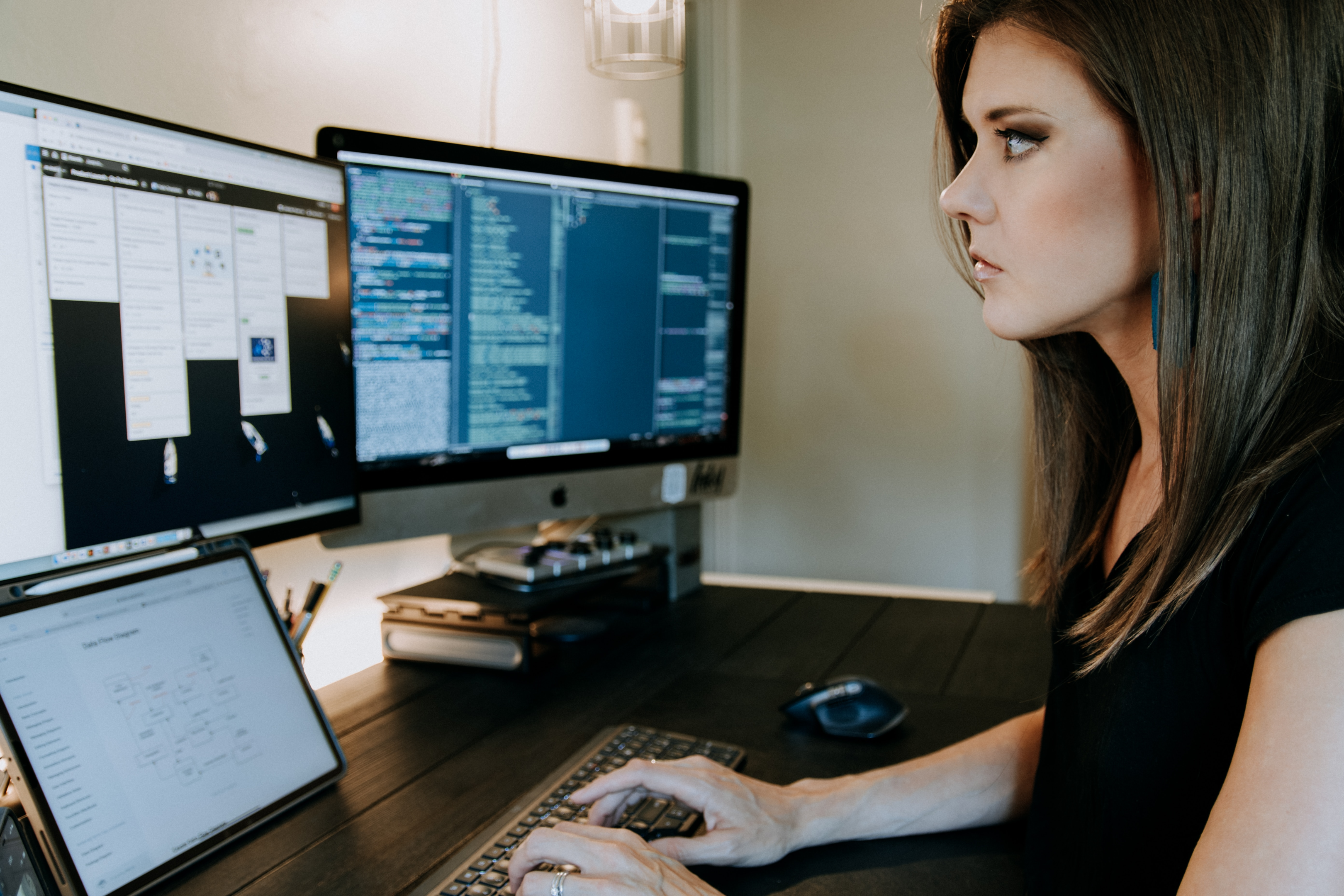
Regression testing plays a critical role in maintaining the quality and functionality of a product. However, we need to outline that running all our regression tests manually when there is space for test automation can be tedious and inefficient.
Don’t miss this article to find out the meaning of regression testing and its relevance in agile teams!
So, when a regression test can be automated, why not do so? In this article, we will cover all you need to know how to automate each regression test that is possible, and how to make your automated regression testing efficient and impactful.
Selection of Test Cases for Regression: How to Choose the Right Tests for Automation
When it comes to automated regression testing, the first step is to select the right test cases. Not all test cases are good candidates for automation. For instance, complex test cases that require human judgment might not be suitable for automation.
On the other hand, test cases that are frequently executed or have been flagged in past test runs could be ideal candidates for automated tests.
Regression Test Selection
Regression test selection involves prioritizing test cases based on specific criteria such as:
Critical functionality: Opt for test cases that cover core features of the software.
When you’re contemplating which test cases to automate for regression testing, your gaze should immediately fixate on the system’s critical functionality.
These are the areas where a failure could have severe implications, affecting both the user experience and the business objectives. Functional testing can often help identify these core features during the initial development phase. Opt for automating these test cases as they are generally stable and not subject to frequent changes.
Frequency: Choose test cases that are often executed.
Frequent execution is another vital factor in the decision-making process. Test cases that are run often can save you a considerable amount of time and resources if automated. These are the test cases that your team would typically perform in manual testing environments.
By automating these tests, you’re not just saving time; you’re also enhancing accuracy since the scope for human error is greatly reduced.
Data sensitivity: Select tests where specific data conditions are crucial.
In some scenarios, the test data plays a pivotal role. These could be test cases where particular data conditions are crucial for the software to function correctly. For example, e-commerce checkout processes or financial transactions.
In such cases, automating regression tests can help in verifying that these specific data conditions are always met.
Advanced Tips for Test Case Selection
- Functionality Over Form: While it might be tempting to automate visually complex and interactive test cases, remember that automated tests excel in verifying functionality over form. Automated tools might not capture nuances that a human eye would. Therefore, complex visual test cases are often better suited for manual tests.
- The Role of Examples: Utilize regression testing examples from past experiences, whether from your organization or external case studies. These examples can act as a blueprint and save you from pitfalls while adding a layer of reliability to your test selection process.
- Regression Testing is Performed in Tandems: Don’t forget that regression testing is often performed in tandem with other types of tests. For instance, you might perform functional tests manually to verify new features, followed by an automated regression test suite to ensure existing functionality is not broken. This tandem approach provides a safety net, ensuring that both new and existing features are functioning as expected.
- Test Automation: The Final Frontier: Once you’ve selected the appropriate test cases for regression testing, the next step is test automation. Various tools can be employed to convert your manual tests into automated scripts. Make sure to choose a tool that fits the language and framework your software is built on. This will not only make the automation process smoother but will also make the maintenance of these test scripts easier in the long run.
By thoughtfully selecting your test cases for automation, you set the stage for a more efficient, accurate, and reliable regression testing process. This selection is a critical component of software quality assurance and plays a substantial role in the software development lifecycle.
In this way, you’re not just automating for the sake of automation but making strategic choices that benefit the software, the development team, and ultimately, the end-users.
What are the benefits of automated functional testing for businesses? Read more here!
Test Case Prioritization in Software Testing
Once you’ve shortlisted the relevant test cases, prioritize them. Some tests may be dependent on the execution of others, or some might be more critical in ensuring the software’s functionality.
Test case prioritization helps in performing regression testing more efficiently by running the most important tests first.
Building Your Automated Regression Testing Strategy
Effective planning and design are pivotal for successful automated regression testing.
Here are some methodologies and tools you can use.
BDD (Behavior Driven Development)
BDD allows both technical and non-technical stakeholders to understand test scenarios. Writing test scripts based on behavior rather than technical aspects fosters clearer communication between the testing and development teams.
TDD (Test Driven Development)
In TDD, writing test cases comes before the code. This ensures that your code changes are always in sync with the existing functionality, making your regression testing process more manageable.
Tools and Software
Selecting the right regression testing tools is a crucial step in automated regression testing.
Popular software testing platforms like Selenium, JUnit, and TestNG have made a significant impact in this field. These tools not only facilitate the writing of test scripts but also integrate well into CI/CD pipelines.
Selenium
Selenium shines when you have to automate regression testing for web-based applications. Its ability to support multiple languages and browsers is a big plus. You can write test cases in Python, Java, or C# and run them on different browsers to ensure the existing functionality is not broken.
JUnit
If your development team works predominantly with Java, JUnit is a sensible choice. This tool integrates well with Maven and Jenkins, making it easier to plug into your CI/CD pipeline. With JUnit, you can handle both unit tests and regression test cases, offering a comprehensive testing suite for your software development life cycle.
TestNG
For those who are looking for a tool that offers more than just unit testing, TestNG is worth considering. It provides functionalities for parallel testing, which is a great way to make your automated regression tests more efficient. It also allows you to prioritize test cases, a feature that comes in handy for test case prioritization in your regression test suite.
By choosing the right tool, your testing team can focus more on creating effective test cases and less on wrestling with software. But remember, no tool is a one-size-fits-all. Assess your project requirements, existing test cases, and long-term goals before making a decision.
Take a look at this article, if you are interested in picking the right tool for automating tests.
Automating Specific Test Cases: A Step-by-step Guide
Once you’ve sorted your test suite, it’s time to start automating your test cases. Let’s dive into a practical example using Selenium as our automation tool.
Writing a Test Script
Writing a test script is the first step in creating automated regression test cases. Using Selenium’s WebDriver, you can easily capture test objects and script out complex scenarios.
import webdriver driver = webdriver.Chrome() driver.get("http://www.yourwebsite.com")
driver.find_element_by_id("login").click()
Validation
The final step in automated test cases is validation. Check whether the test cases pass or fail based on your expectations. Failed test cases need to be scrutinized to see if it’s a bug fix or an issue with the test script itself.
if "Dashboard" in driver.title: print("Test passed") else: print("Test failed")
Test Execution – Perform Regression Testing
After writing the test scripts, the next step is to execute them. Automated tools like Selenium make it easy to run regression tests. Make sure your testing environment is set up correctly before test execution.
Optimizing Regression Tests
Performing regression tests can be time-consuming, but various regression testing techniques and parallel testing can speed up the process.
Parallel Testing
One method to optimize your regression testing is to perform parallel tests. This enables you to run multiple test cases at the same time, thereby saving a lot of time. Parallel testing works best when you’re looking to perform regression tests across different configurations and environments.
Code Changes and Retesting
When there are minor code changes, it’s not necessary to run all the test cases. Use test case prioritization to select only those test cases that are relevant to the areas impacted by the code changes.
Handling Test Data
Managing test data effectively is crucial in automated regression testing.
Data Setup
Before running your regression tests, make sure you have all the necessary test data in place. The data should be as close as possible to the data that will be used in the production environment.
Data Validation
Post-execution, validate your test data to ensure that your automated testing has covered all scenarios. Data validation helps in identifying any issues with existing functionality or new features added.
Integrating Regressions into CI/CD Pipeline Automated Testing
The beauty of automated regression testing shines when integrated into a Continuous Integration/Continuous Deployment (CI/CD) pipeline. A CI/CD pipeline automates steps in the software delivery process, such as initiating code builds, running automated tests, and deploying to a staging or production environment.
The integration of automated regression testing into a CI/CD pipeline is a leap toward more efficient software development. By doing so, you can ensure that regression tests run automatically with every code change, maintaining the software’s existing functionality intact.
Jenkins: A Go-To for Many
Jenkins is a popular tool for setting up CI/CD pipelines. It’s extremely versatile, supporting a multitude of plugins and integrations, making it suitable for almost any testing method or framework you might choose.
Jenkins not only triggers automated regression tests as soon as code changes are committed but also provides detailed reporting features. These reports can help in analyzing test cases that have failed and are invaluable for continuous improvement in automated testing methods.
GitHub Actions: Simplicity and Ease
If your source code is hosted on GitHub, then GitHub Actions might be the simplest way to automate your regression testing process.
It allows you to define your automation testing workflows using YAML files stored in your GitHub repository, making it straightforward to set up, inspect, and manage your automated regression testing.
GitLab CI/CD: All-in-One
GitLab’s built-in CI/CD service is another excellent choice for automating regression tests. Similar to Jenkins and GitHub Actions, GitLab CI/CD can be triggered automatically whenever you commit or push changes.
Its built-in Docker support means you can also run tests in a containerized environment, perfect for ensuring that test cases run the same way wherever they’re executed.
Monitoring and Feedback
Once automated tests are up and running in your pipeline, it’s crucial to set up monitoring and feedback loops. Monitoring helps in quickly identifying what went wrong, while feedback loops ensure that the development team is informed promptly. Both are essential to ensure that regression tests are adjusted and improved over time.
Incorporating automated regression testing into your CI/CD setup ensures that you are constantly performing regression tests on the latest version of the application, minimizing risks and improving software quality.
Reporting and Analyzing Results – Complete Regression Testing
Once your automated regression tests are integrated into the CI/CD pipeline and executed, the next pivotal step is understanding the results.
Analyzing test results is essential for making informed decisions about the application’s quality, identifying areas for improvement, and planning future tests.
Understanding Test Reports
Your team should be equipped to read and interpret the output provided by regression testing tools. Most modern tools offer detailed reports, including the number of test cases executed, passed, and failed.
Diving deep into failed test cases can offer insights into whether a bug fix was successful or if the latest code changes have introduced new bugs.
Use of Analytics Tools
Tools like TestRail and QMetry offer analytics features that allow teams to create custom dashboards. These dashboards can display key performance indicators such as test execution time, failure rates, and more.
The ability to visualize this data can be incredibly helpful for both the development team and stakeholders.
Continuous Feedback for Improvement
The outcome of regression tests should not just be a checkmark in your testing process but should serve as a constant feedback mechanism. Continuous feedback is crucial for improving both the automated regression testing process and the product itself.
Future Trends in Automated Regression Testing
As we continue to evolve in the software testing landscape, staying ahead of future trends is pivotal. Here’s what to look out for:
Artificial Intelligence
One trend that’s impossible to ignore is the role of Artificial Intelligence in automated testing. AI algorithms can now predict which areas of the application are most likely to fail, enabling test case prioritization.
More Cloud-based Solutions
With the widespread adoption of cloud computing, we can expect more cloud-based automation testing solutions. These solutions will make it easier for remote teams to collaborate and perform regression testing simultaneously.
Integration of Big Data
With big data, the focus will be on analytics and how to use vast amounts of test data for improving test case selection and testing methods.
The Bottom Line
The seamless integration of automated regression testing into a CI/CD pipeline serves as a cornerstone for enhancing the software development process. By doing so, it enables organizations to maintain a continuous focus on quality while rapidly adapting to changes.
The automation tools, while significant, are mere enablers in this equation. The crux of the strategy lies in the synergy between the development and testing teams, their shared vision, and the mutual commitment to ongoing improvement.
Proper planning and a well-defined test strategy are vital. The goal is not just to run tests but to run meaningful tests that provide valuable insights. Knowing how to set up, interpret, and act on these tests is equally as crucial, as it’s this knowledge that transforms raw data into actionable insights for future developments and refinements.
Monitoring and feedback mechanisms are also indispensable. They serve to provide real-time awareness and insights into the testing process, allowing for immediate adjustments and long-term refinements. These cycles of feedback and improvement not only optimize the testing approach but also enhance the overall quality of the product.
In summary, while integrating automated regression testing into a CI/CD pipeline may not offer an absolute assurance of faultlessness, it does create an environment where quality can be consistently pursued and enhanced.
The ability to implement and manage this process proficiently is crucial, positioning it as an integral element in the modern software development ecosystem.
Looking for a Quality Assurance Partner for Automating Regression Tests?
We are quality partners! Start regression testing as part of an automated functional testing strategy today! Contact us to discuss how we can help you grow your business.

Tags In
Related Posts
Selenium vs Watir
Choosing between these two test automation tools for an automation framework I recently got started on a client project in which I’m putting together an automation framework, where, luckily, I’ve been given some objectives that are quite clear to fulfill. I want to discuss one…
[Ebook] A Complete Introduction to Functional Test Automation
Everything you need to know before getting started with functional test automation Introduction to Functional Test Automation It is often said that “Automating chaos just gives faster chaos,” and not only faster, but also (paraphrasing a Daft Punk song) harder, faster, stronger… chaos. Yet, seemingly everyone…
Search
Contents