Chances are you’ve heard the term API. However, you might not know exactly what it is, similar to the mystification of the “cloud” in the early 2000s. In this post, I will break down what an API is, using a familiar example, and how to go about testing it. By the end of this post, you won’t just be familiar with the term API, you’ll be driving the API testing strategy for your organization.
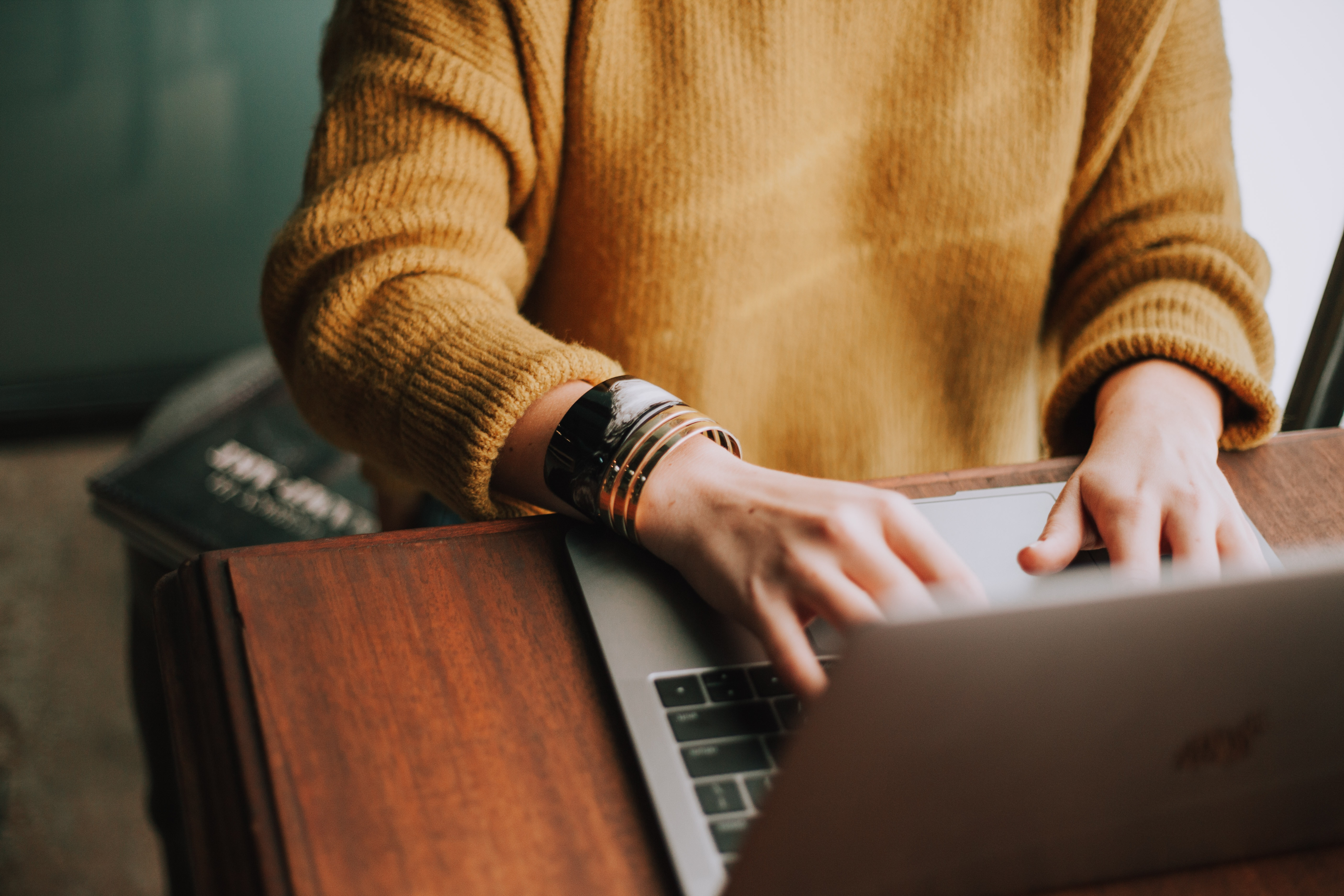
What is an API?
Merriam-Webster defines API (Application Programming Interface) as “a set of rules that allows programmers to develop software for a particular operating system without having to be completely familiar with that operating system”. Said differently, you don’t need to know everything about the system you’re trying to interact with. The creators of the API will define everything for you, such as what data can you send them (requests) and what data you can get back (responses).
Let me share an example using the Apple Maps “Ride” option. If you’re on your iOS device and you want to get a Lyft ride to the destination you’re searching for, you simply need to hit the “Ride” option. Instead of having to go out of the Apple Maps application and into Lyft, Lyft provides an API so Apple Maps can “call” and get the information it needs to show you ride options.
To break this down a bit more, when you click on the “Ride” option in Apple Maps, Apple has to send Lyft some information in a request so Lyft knows what information (response) to send back. This information might be, “Where exactly are you” and “Where do you want to go”. That request is sent to Lyft. Lyft reads it and sends back a response with the information requested. The information being requested by Apple Maps would probably be “What types of cars are available”, “how long until a car shows up at the starting point” and, “How much will it cost for each ride option”. Apple’s front end (User Interface) is set up to gather the response and show you the results in a usable manner.
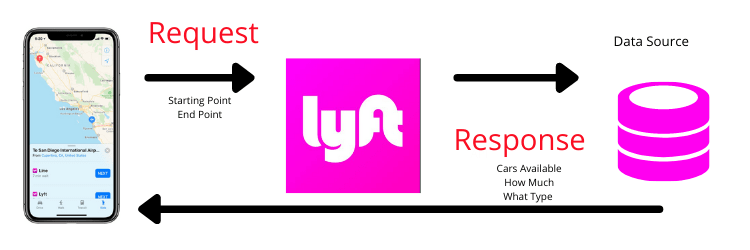
API Testing Types and Their Purposes
Functionality: The purpose of functional testing is to ensure you can send a request and get back the anticipated response using the requirements the API outlines. This includes negative and positive testing.
- Ensure you’re covering all of the possible combinations of data. Don’t forget negative and positive testing!
- Do not rely on UI testing for this. There could be bugs rooted in the unit level or backend that wouldn’t be visible via UI testing.
- Error handling when data is outside of accepted parameters must be considered.
Security: The purpose of security testing is to make certain your communication with the API is secure and the person making the call is only allowed to do what they’ve been permitted to do.
- Validate that your data is encrypted appropriately.
- What type of authentication is required? Said another way, are you who you say you are?
- What type of authorization should you have? In other words, are you allowed to do what you’re requesting to do?
- Validate OAuth 2.0 workflows used to secure API calls:
- Sending tokens, you can test the authorization and authentications from above.
Performance: The purpose of performance testing an API is to ensure it can handle user load and determine what happens when it reaches that load limit.
- Increase the number of API calls and then monitor response times and throughput.
- Monitor for memory leaks by performing an endurance test.
- Stress the system out by loading it down with calls – how does it respond to failures and breakage?
Reliability: The purpose of reliability testing is to find possible interruptions. What happens if connectivity goes down in the middle of the test?
Documentation: The purpose of reviewing documentation is to validate that the documentation provides enough information to interact with the API. This is typically done as you’re testing the API. Do you have the information you need to successfully execute the testing? Would someone consuming this information know how to interact with the API?
Integration Testing: The purpose of integration testing is to verify success where multiple APIs are working together. Focus on call sequencing and ensure data is returned promptly and accurately.
Types of API Protocols
While there are multiple types of API protocols, I’ll focus on a couple of the most common ones for a quick overview.
- REST: The most common API used today, it’s a web service API and stateless in nature. REST uses multiple standards such as HTTP, JSON, URL, and XML.
- SOAP: One of the original protocols, it is used less often because of its strict standards. SOAP uses HTTP and XML standards.
API Actions
These are the various actions you can perform when API testing:
- GET: This is the most common action and a way to simply tell the web service that you want to retrieve, or get, some information.
- Use heuristics to ensure you’re not just checking sunny day scenarios
- DELETE: This action will allow you to delete something.
- Make certain to test that you can’t delete things you shouldn’t be able to. On the flip side, can you delete what you’re given authorization to?
- POST: This action will tell the server that you want to create something new. For example, if you want to create a new product you could use this action. To do so, you’d have to input parameters so it knows what you want to create.
- Ensure malicious users cannot intentionally cause harm OR unknowingly cause harm. This might include sending bad or missing parameter data like required fields.
- PUT: This action will perform an action on something existing, like updating an existing product. Similar testing strategies might be employed between PUT and POST calls.
Preparing to Test an API
Before you start executing your testing, you’ll want to ensure you have outlined some testing parameters and fully understand the API:
- Does this API interact with other APIs?
- What User flows would trigger the API calls?
- Get the documentation for the API so the features and functions are understood.
- Outline positive and negative scenarios for input parameters.
- What are the API response requirements?
- What are the typical API loads? Work with your Product Manager to understand these metrics.
- Make certain you have a test environment in which to test the API.
Testing an API
Testing an API is as simple as submitting a request on behalf of an application (Client), using another application’s API (Server), and checking that it returns the expected response. While that seems straightforward, there can be a learning curve. The first, and greatest learning curve, is the tooling. To test an API, you’ll need to select an API testing tool to use. I will cover those options below. For now, let’s look at how to execute a non-automated, sunny-day scenario, using Postman.
Step 1: Enter the URL (using the GET function) and define the parameters. In whatever tool you choose, you’ll need to tell it what API you want to test. In addition, you may need to define parameter values. If you’re looking for one to play with, here you go: https://jobs.github.com/positions.json?description=python&full_time=true&location=sf. You can define the value for each parameter to play around with. Using that API, you can see I have three parameters I can define: description (value of “python”, full_time (value of true), and location (value of sf). If I were testing multiple variations of these parameters, I would change the values to match my scenarios.
Step 2: Hit Send. You want to send your request, with specific parameters (the input), to the API.
Step 3: Review the response status. If you’re expecting no issues, you’d be looking for a response of 200.
Step 4: Review the response time. Determine what your performance limits are and ensure it returns within that threshold.
Step 5: Review the response body. Ensure the response body matches the expected results.
Step 6: Review the response headers. Ensure the response header matches the expected results.
API Testing for Mobile
API testing for mobile can be done similarly to web API testing with the appropriate testing tool such as Postman (see the section on tools).
Best Practices and Random Advice
- Parameters to be used during testing should be called out in the test case.
- Be careful if you’re using the Delete or Purge functions – those are one-time calls.
- If you’re testing multiple APIs, consider the call sequencing.
- Start with outlining all test scenarios before beginning; group the tests accordingly.
- Make sure you look at similar APIs. Does this API follow modeling best practices? I’ve experienced an example where there were two different APIs that should call the same data. One for the current day and the other for previous time periods. The response content for each was considerably different – not good.
Challenges of API Testing
- Documentation should be standard for any organization using APIs. However, that isn’t always the case. Without proper documentation, you can’t adequately test.
- Being able to understand and write scenarios for all of the parameter combinations and call sequencing is tough. Especially if you get a large API with many parameter options intertwined with other APIs. In this case, I would use something like Equivalence Partitioning Testing to avoid over-testing and a Decision Table to ensure I hit all the combinations.
- Learning an API testing tool can be challenging. Thankfully there are tons of YouTube videos of people eager to help. More information on that in the next section.
- Exception and error handling are both challenging, as those things are rarely defined for you. Work with your dev team to understand what those exceptions or errors might be.
- It’s intimidating. API testing is technical which causes some people to shy away from doing it. Start with basic testing. Use basic parameterization using simple GET calls. Work your way forward from there.
API Testing Tools and How to Resources
I was all hunkered down to outline all the testing tools I’ve used or heard good things about. And then, the Twitter Gods bestowed upon me a list of the top 10 API tools by Testim, which was just updated and re-published in early 2021. Instead of reinventing the wheel, I’ll link you to their article on it: https://www.testim.io/blog/the-9-api-testing-tools-you-cant-live-without-in-2019-2/
My personal tool of choice is Postman. It’s what I learned and I’m comfortable it can handle everything I throw at it.
Additionally, I want to share some personal links to places that I’ve learned quite a bit from over time. I’m dollar-conscience so these are all FREE learning opportunities. You’ll find everything from learning about API testing to learning specific tools you might choose.
- Restful Booker: This is a website that hosts several APIs, with documentation, so you can play around and learn more. You can create, read, update and delete on this! https://restful-booker.herokuapp.com/
- LinkedIn: LinkedIn will give you a 30-day free trial to their learning platform. It would be worth signing up to take advantage of all the courses they have on API Testing. https://www.linkedin.com/learning/search?keywords=API%20testing
- If you’re going with Postman, you have to check out this GitHub repo. Danny Dainton captures “All Things Postman”. https://github.com/DannyDainton/All-Things-Postman
- If you get stumped and need some real-time help, the Ministry of Testing has a free slack world you can join and a dedicated #api-testing channel that stays pretty active. https://www.ministryoftesting.com/slack_invite
Conclusion
I truly hope this API testing guide has helped you get a better grip on this aspect of software testing. For so many, API testing remains an overwhelming and intimidating testing phase. Use the resources I’ve provided you, pick out a tool that looks best for you, start practicing, and in no time you’ll be more confident in your API testing.
Let me know in the comments, are you starting to learn more about API testing? Any other tips and resources that you would add?
About the Author
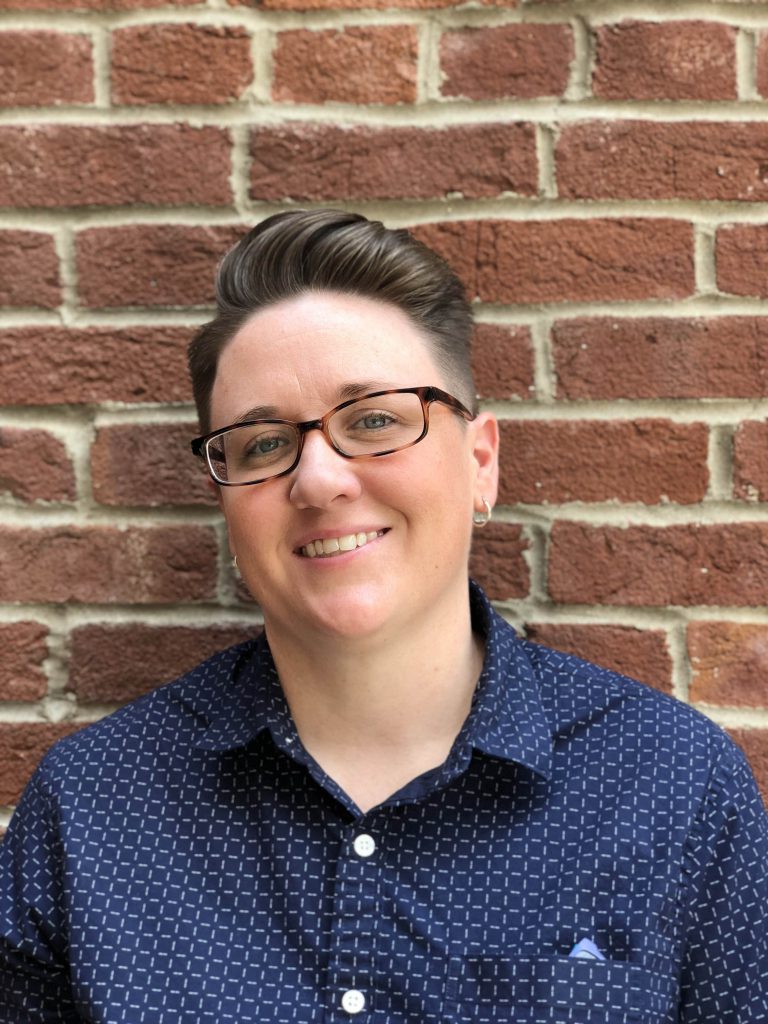
Johanna South has been actively testing or evangelizing software testing concepts for a little over a decade. She has worked in various roles throughout the Software Development Lifecycle, including manual and automation testing, systems analyst, and leading full-stack software development teams. Her broad understanding of delivering quality software gives her a unique perspective in which to pull ideas. Johanna is currently a Sr. Director of QA for TwinSpires.com.
Check out more of her articles at theqaconnection.com.
Related Posts
[Infographic] 6 Software Testing Outsourcing Myths Debunked
Putting to bed your fears about outsourcing software testing and QA once and for all Have you turned your back on the idea of outsourcing your software testing and QA (what we prefer to call quality engineering)? Maybe it was brought up once during a meeting…
Code Analysis Part 2: Analyzing Code with SonarQube
Continuing with our code analysis series, here’s an introduction to SonarQube As we mentioned in part 1 of this 3 part series on code analysis (on what you should know about technical debt), code quality is often said to be an internal attribute of quality, since…
Search
Contents